How to Build an Open Source AI Agent for Code Scanning
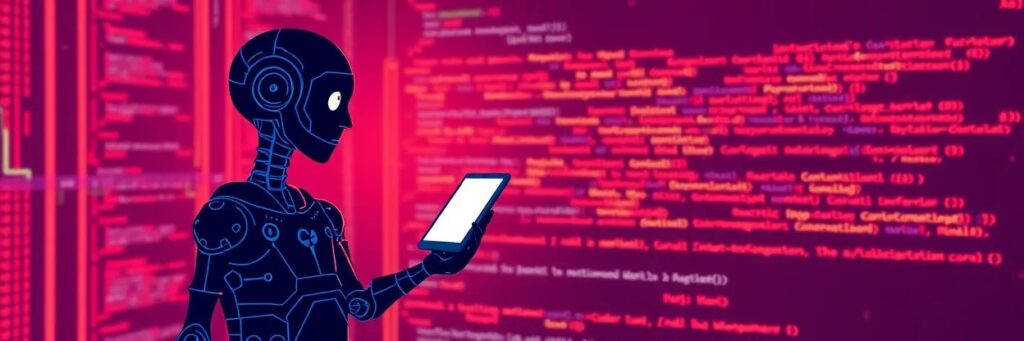
Image Source: opportunitymentor
In today’s rapidly evolving software landscape, ensuring code quality and security is paramount. This guide will teach you how to build an open source AI agent for code scanning that automates the process of identifying vulnerabilities and code issues. Although many static analysis tools exist, combining artificial intelligence with open source frameworks offers a smarter, adaptive approach to code scanning.
Why Build an AI Agent for Code Scanning?
Traditional code scanning tools work well for routine checks; however, they often struggle with evolving code patterns and complex vulnerabilities. In contrast, an AI agent can learn from new data, adapt to emerging threats, and provide more nuanced insights. Consequently, using an open source approach allows developers to customize and improve the scanning process continuously.
Prerequisites
Before you start, ensure you have the following in place:
- Familiarity with Python: Although this guide focuses on AI and code scanning, Python’s ecosystem is ideal for prototyping machine learning models.
- Basic Machine Learning Knowledge: Understanding fundamental ML concepts will help you fine-tune the agent.
- Development Environment: Install Python (3.8+), and set up a virtual environment.
Step-by-Step Guide
1. Define Your Objective
First, clearly determine what you want your AI agent to do. For instance, you may want it to scan source code for common vulnerabilities such as SQL injection, unsafe use of eval
, or other risky patterns. By defining the scope, you can better tailor your model and rules.
2. Choose Your Tools and Frameworks
There are several open source libraries available that can help you build an AI agent for code scanning. For example, you can leverage Hugging Face’s transformers along with CodeBERT to analyze code snippets. Additionally, you might integrate traditional static analysis tools for baseline checks.
Example: Using CodeBERT for Code Analysis
from transformers import AutoTokenizer, AutoModelForSequenceClassification import torch # Load a pre-trained model (CodeBERT) tokenizer = AutoTokenizer.from_pretrained("microsoft/codebert-base") model = AutoModelForSequenceClassification.from_pretrained("microsoft/codebert-base") def scan_code(code): inputs = tokenizer(code, return_tensors="pt", truncation=True, max_length=512) outputs = model(**inputs) probs = torch.softmax(outputs.logits, dim=1) # Assume index 1 represents the vulnerability class vulnerability_score = probs[0][1].item() if vulnerability_score > 0.7: return "Potential Vulnerability Detected" else: return "Code Appears Safe" code_sample = "eval(user_input)" print(scan_code(code_sample))
In this snippet, we load CodeBERT to predict whether a given piece of code might be vulnerable. Although this is a conceptual example, it demonstrates how you can combine open source models with custom logic to build your AI agent.
3. Prepare and Label Data
Next, collect and label your dataset. You can use public repositories, open source projects, or your own codebase to gather examples. It is essential to label the data—identifying which code snippets are safe and which contain vulnerabilities—so that the model can learn effectively.
4. Train Your Model
Now, train your model using the labeled dataset. Depending on your requirements, you can fine-tune a pre-trained model such as CodeBERT on your specific data. This will allow the AI agent to adapt to the unique code patterns in your projects.
5. Integrate with Code Scanning Tools
Additionally, consider integrating your AI agent with existing code scanning tools (such as static analyzers) to enhance its accuracy. For example, you can combine the AI’s predictions with traditional rules-based checks.
import re def traditional_scan(code): vulnerabilities = [] if "eval(" in code: vulnerabilities.append("Usage of eval detected") # Add more rule-based checks as needed return vulnerabilities sample_code = "eval(user_input)" print(traditional_scan(sample_code))
In this example, a simple regular expression check is combined with AI predictions for a robust scanning solution.
6. Test, Debug, and Iterate
Testing is crucial. Validate your AI agent with both synthetic and real-world code samples. Use debugging tools and monitor its performance to iteratively improve accuracy. Moreover, continuous feedback is key to refining your model.
7. Deploy Your AI Agent
Finally, deploy your AI agent into your development pipeline. You can integrate it with your CI/CD tools, web applications, or use it as a standalone CLI tool. Hosting platforms such as Heroku or AWS can make deployment seamless.
Challenges and Solutions
Although building an AI agent for code scanning is promising, it comes with challenges. Below is a brief table outlining common challenges and their solutions:
Challenge | Solution |
---|---|
Limited Training Data | Augment your dataset using public repositories and synthetic data generation. |
Integration Complexity | Combine AI predictions with traditional static analysis for a comprehensive solution. |
Performance Bottlenecks | Optimize your model inference and consider scalable cloud hosting solutions. |
Real-World Examples
To illustrate the concept, consider these examples:
- Vulnerability Scanning: An AI agent that detects insecure coding practices and potential exploits in source code.
- Code Quality Analysis: A tool that reviews code for maintainability, code smells, and adherence to style guidelines.
Conclusion
In summary, building an open source AI agent for code scanning empowers developers to automate and enhance code analysis processes. By leveraging state-of-the-art open source models, integrating them with traditional scanning techniques, and continuously refining your approach, you can create a robust solution that improves code quality and security. Ultimately, the journey involves experimentation, iteration, and community collaboration.
We hope you found this guide insightful and practical. Happy coding, and may your code always be secure!