Step-by-Step Guide: How to Build AI Agents with Ruby
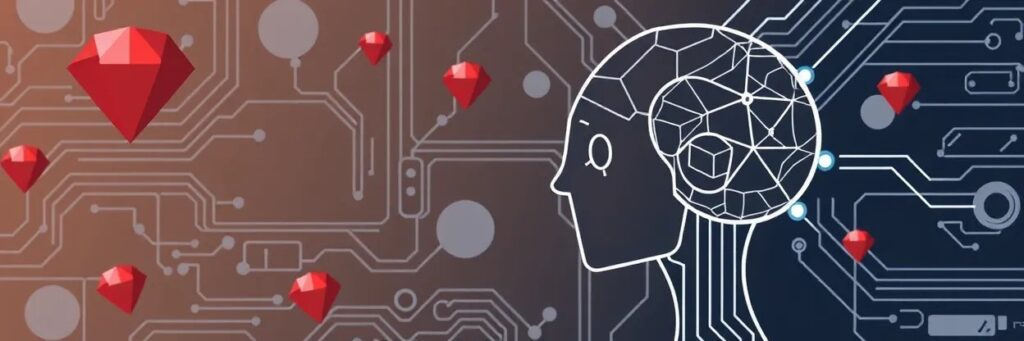
Image Source: opportunitymentor
Artificial Intelligence (AI) agents are transforming industries by automating tasks, learning from data, and making real-time decisions. While Python dominates AI development, Ruby’s simplicity and readability make it an excellent choice for building lightweight yet powerful AI agents. In this guide, we walk you through a technical, step-by-step process—complete with code examples and best practices—to build your own AI agents with Ruby.
Why Ruby for AI Agents?
Ruby may not be the first language that comes to mind for AI development, but it has many advantages:
- Beginner-friendly syntax: Ruby’s clean and concise code structure simplifies prototyping.
- Robust Ruby gems: Libraries such as
ruby-ml
,Numo::NArray
, andTensorFlow.rb
empower numerical computing and machine learning. - Rapid development: Ruby’s object-oriented design accelerates the implementation of complex agent logic.
- Active community: Extensive support via forums and documentation helps streamline troubleshooting.
Prerequisites
Before you begin, ensure that you have the following installed on your system:
- Ruby (version 3.0 or higher) and RubyGems
- Key Gems: Run the following command to install the necessary libraries:
gem install numo-narray sciruby ruby-ml regent
Step-by-Step Guide
1. Define Your Agent’s Purpose
AI agents serve a variety of use cases. For instance, they can act as chatbots for customer support, power recommendation systems, or even detect fraudulent activities. As an example, you might build a weather-prediction agent using historical data.
2. Choose a Framework or Library
Depending on the complexity of your project, you can select from several frameworks. Here are two options:
Option 1: Ruby-ML for Simple Models
require 'ruby-ml' # Sample dataset: [Outlook, Temperature] => Decision (Yes/No) data = [ ['Sunny', 'Hot', 'No'], ['Overcast', 'Hot', 'Yes'], # ... (14 rows) ] features = data.map { |row| row[0..1] } labels = data.map { |row| row[2] } # Train a decision tree tree = RubyML::Classification::DecisionTree.new tree.train(features, labels) # Predict test_data = [['Overcast', 'Cool']] puts tree.predict(test_data) # Expected output: ["Yes"]
As you can see, Ruby-ML provides a simple way to train a model and make predictions.
Option 2: Regent Framework for Advanced Agents
# Define a weather agent using Regent class WeatherAgent < Regent::Agent tool(:weather_tool, "Get weather for a location") def weather_tool(location) "72°F and sunny in #{location}" end end agent = WeatherAgent.new("Weather Assistant", model: "gpt-4o") puts agent.run("Tokyo weather?") # Expected output: "72°F and sunny in Tokyo"
The Regent framework allows you to build more advanced, interactive agents with minimal code.
3. Prepare Training Data
Next, gather and clean your data. Use structured datasets (CSV or JSON) or scrape data via APIs. Ensure that you handle missing values and outliers properly so that your agent learns effectively.
4. Design the Agent’s Workflow
Now, design how your agent will operate. You can choose between:
- Rule-based systems: Predefine logic (e.g., "IF temperature > 30°C, THEN alert").
- Learning-based systems: Train models on historical data so the agent adapts over time.
5. Integrate with External Systems
In many cases, your AI agent will need to interact with external APIs or databases. For example, if you want your agent to track orders, you can integrate with Shopify’s API:
require 'net/http' require 'json' def fetch_order_status(order_id) uri = URI("https://api.shopify.com/orders/#{order_id}") response = Net::HTTP.get(uri) JSON.parse(response)['status'] end puts fetch_order_status(12345)
This code snippet demonstrates a basic API call to fetch order status.
6. Test and Debug
Testing is crucial. Validate your agent’s performance with test datasets and use debugging tools such as Regent’s built-in tracing to understand decision-making processes.
7. Deploy the Agent
Finally, deploy your agent. You can integrate it into web applications using frameworks like Rails or Sinatra, package it as a CLI tool, or host it on cloud platforms such as Heroku or AWS.
Challenges and Solutions
Every approach has its challenges. Below is a table highlighting common issues and potential solutions:
Challenge | Solution |
---|---|
Performance Limitations | Utilize TensorFlow.rb for heavy computational tasks. |
Smaller ML Ecosystem | Integrate Python models via APIs if needed. |
Complex AI Concepts | Begin with rule-based agents before moving to learning-based models. |
Real-World Examples
To put theory into practice, consider these real-world implementations:
- E-commerce Chatbot: Automates FAQs, fetches order statuses, and escalates to human agents when necessary.
- Fraud Detection Agent: Analyzes transactional patterns using anomaly detection methods.
Conclusion
Ruby’s simplicity and its growing ecosystem of AI libraries make it a compelling choice for developers looking to build efficient AI agents. By following this guide, you now have the tools and knowledge to create agents that automate tasks, learn from data, and integrate with external systems. Remember to start small, iterate often, and continuously refine your solution based on feedback.
We hope you enjoyed this step-by-step guide. Happy coding!
Additional Resources
- No-Code AI Agent Builder: A Beginner’s Guide
- AI Agent vs. Chatbot: Understanding the Key Differences
- How to Create Free AI Agents for Your Business
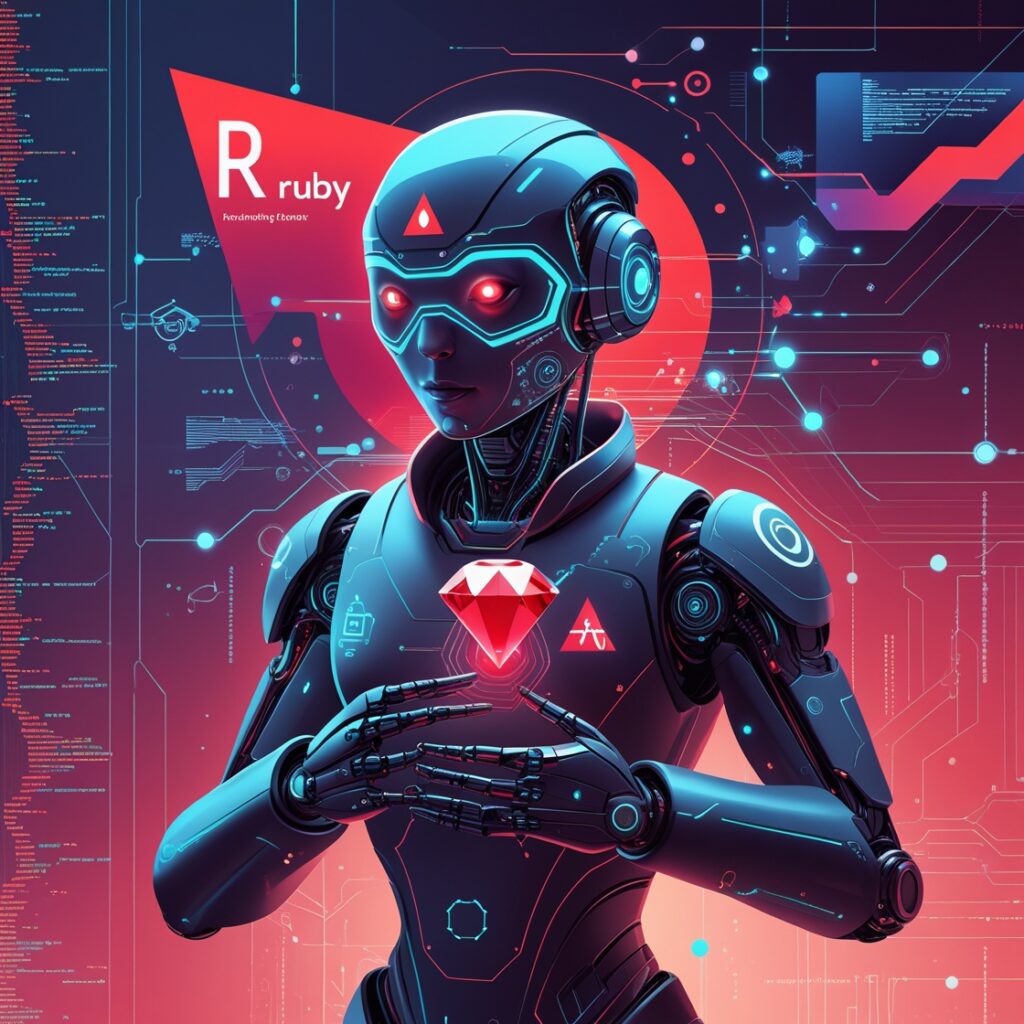